Save Data with AJAX in MVC
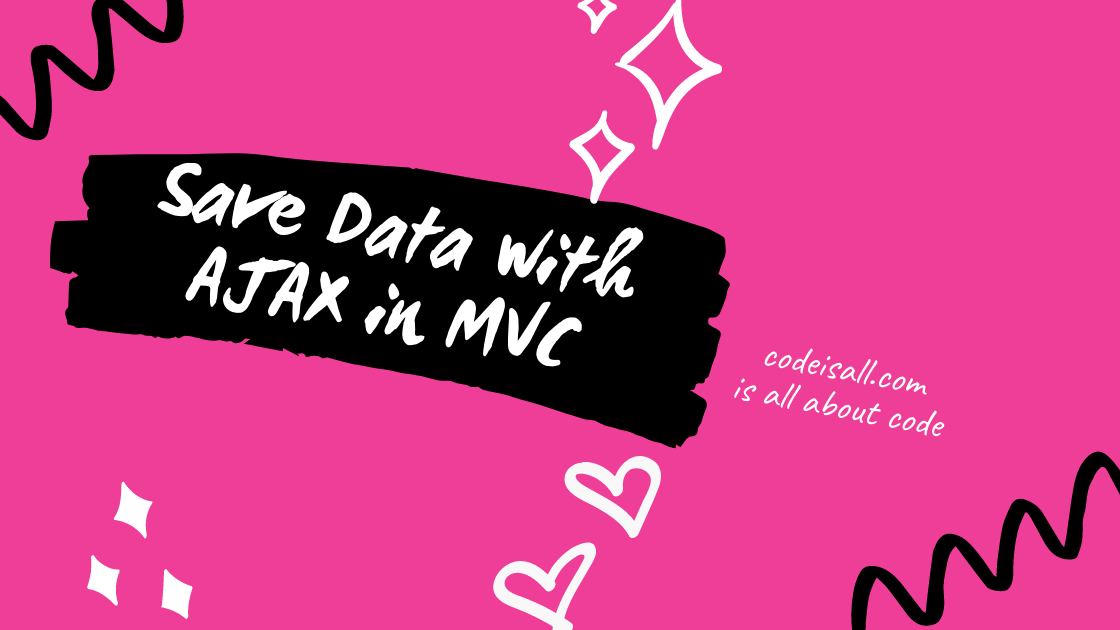
In the previous article, you learned about what is the jQuery Datatables and how we can use them in MVC applications. In this article, you will learn about what AJAX is, the advantages of AJAX request in web applications, and how to use it in an MVC application.
What Is AJAX?
AJAX stands for Asynchronous JavaScript And XML. It is not a programming language, it is just uses combination of browser built-in XMLHttpRequest object, JavaScript, HTML, XHTML, CSS, XML and DOM.
When HTML, CSS, XML, XMLHttpRequest object, and javascript are combined in the Ajax model, web applications are able to make quick updates to the user interface without reloading the entire browser page. This makes the application faster and more responsive to user actions.
Advantages of AJAX
- Update a web page without reloading the page
- Request and Receive data from a server after the page has loaded
- Send data to a server in the background.
- It is a web browser technology independent of web server software.
- Data-driven as opposed to page-driven.
How It Works
AJAX can’t work independently. because of its combinations of other technologies.
JavaScript
- Loosely typed scripting language.
- the function is called when an event occurs on a page.
- Glue for the whole AJAX operation.
DOM (Document Object Model)
- API for accessing and manipulating structured documents.
- Represents the structure of XML and HTML documents
CSS
- Allows for a clear separation of the presentation style from the content and may be changed programmatically by JavaScript
XMLHttpRequest
- JavaScript object that performs asynchronous interaction with the server.
AJAX in .NET MVC
Step 1
Create a new project in asp.NET MVC, you can refer to creating an asp.NET MVC application from here, Either you can also implement it in existing projects as well.
Step 2
Crete view for following example that demonstrates the use of AJAX. write the following code in your view file.
<div class="row justify-content-md-center"> <div class="col col-lg-12 form-group text-center"> <label class="h3"> <b>Basic Probability Calculator</b> </label> <hr> </div> <div class="col col-lg-6 form-group"> <label for="" class="fw-500">Probability 1</label> <input class="form-control form-control-sm number" id="Probability1" placeholder="Enter Probabilty 1" type="number"> </div> <div class="col col-lg-6 form-group"> <label for="" class="fw-500">Probability 2</label> <input class="form-control form-control-sm number" id="Probability2" placeholder="Enter Probabilty 2" type="number"> </div> <div class="col col-lg-12 form-group"> <label for="" class="fw-500">Function</label> <select class="form-control form-control-sm" id="Function"> <option value="">Select function</option> <option value="Combined_With">Function 1</option> <option value="Either">Function 2</option> </select> </div> <div class="col col-lg-6 form-group"> <label></label> <label class="text-info m" id="lblResult"></label> </div> <div class="col col-lg-6 form-group"> <label></label> <button type="button" class="btn btn-primary pull-right" id="btnCalculate">Calculate</button> </div> </div> @section Scripts{ <script> $("#btnCalculate").on("click", function () { var calculatorModel = { "Probability1": $("#Probability1").val(), "Probability2": $("#Probability2").val(), "Function": $("#Function").val(), } $.ajax({ url: '@Url.Action("CalculateProbability", "Calculator")', type: 'POST', data: calculatorModel, success: function (result) { $("#lblResult").text("Probability Result: "+result.result); } }); }); </script> }
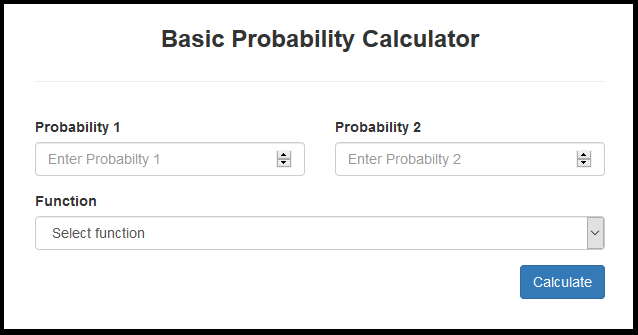
In the following code, there is two input box for inputting two probability values from the user and one dropdown for selecting the probability method for calculation.
In the script section button click event written for request calculation of two user inputs from server. first, it will get data using the id selector from both input fields and dropdown and wrap it into calculatorModel. you can also pass separate value to the server parameters if you want.
AJAX function is called when a button is clicked and the js model bind from user inputs. you can check there are various options for AJAX functions like URL, type, data, async, dataType, etc. you can find all options from here.
- URL: a string that contains address where request is sent.
- TYPE: The HTTP method to use for the request, by default is GET. you can set it to PUT, POST, etc.
- DATA: data that is sent to the server, is in form of a Plain Object String, or Array. if you are using the HTTP GET method then you can append data at the end of URL.
- SUCCESS: A function to be called if the request succeeds. here if request succeeds then text set in result div.
Step 3
Create one model that contains the property of two probability values and the method of probability calculation.
namespace ProbabilityCalc.Models { public class CalculatorModel { public decimal Probability1 { get; set; } public decimal Probability2 { get; set; } public string Function { get; set; } } }
Step 4
Write method in the controller for calculating probability from user input and send the response as JSON format.
[HttpPost] public JsonResult CalculateProbability(CalculatorModel calculatorModel) { decimal Result = 0; if (calculatorModel.Function == "Combined_With") Result = calculatorModel.Probability1 * calculatorModel.Probability2; else Result = (calculatorModel.Probability1 + calculatorModel.Probability2) - (calculatorModel.Probability1 * calculatorModel.Probability2); return Json(new { Result }); }
the following code get user value from request model and calculate based on the selected model and return the calculated value as a response. this response is displayed in the AJAX success function.
you can also add some validation to prevent failure from the server. you can add javascript validation from client-side or user server-side validation.
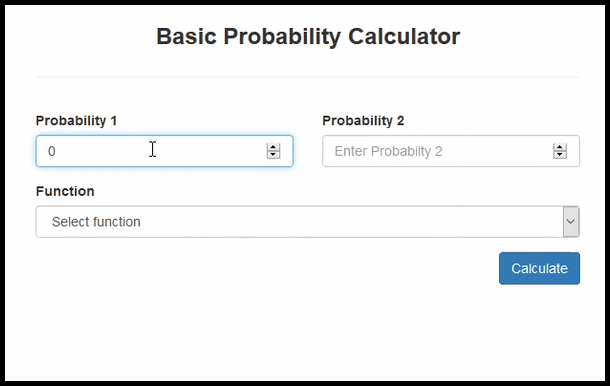
As shown in the output user can send a request to the server and get a response from the server without the page reloading. you can use AJAX to save data to the database, get data from the server, and many more.
I hope this article will help you to understand how AJAX request is useful in web applications how can be used in MVC applications and also what is the advantages of jQuery Datatables. We will be happy to help you if you have any issues or queries. Comment your suggestions and issues and we will try to resolve it.