Cookies & Session in ASP.NET MVC
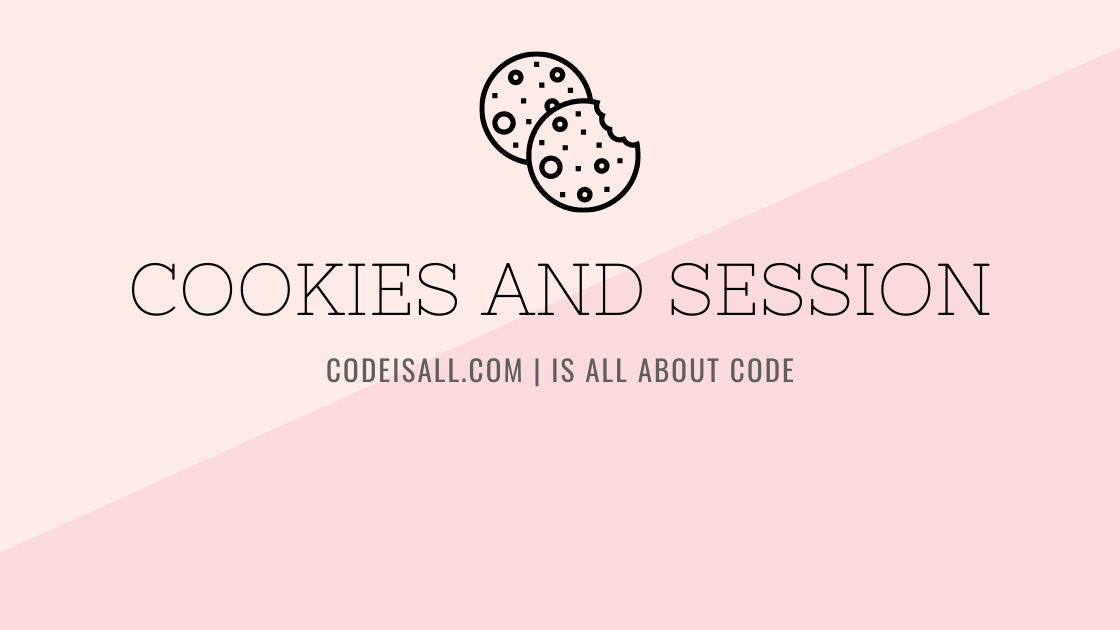
Introduction
In the previous article, you learned about what is Bundling and Minification in the MVC applications.
In this article, you will learn about cookies and sessions and how to use this in an MVC application.
This article will be helpful to beginners and students who are learning.
Cookies
Cookies are used to store information. It is stored only on the client-side machine. Basically, cookies are text files stored on a client machine for tracking purposes.
The server sends a set of cookies to the browser. For example age, name or gender, identification number, etc. And browser saves this information on a local machine for future use.
So, when the browser sends a request to the server, it sends the cookies along with the request, so that server can identify which user sent this request.
Cookie store in client-side storage of data variables. Different users at the same machine can read the same cookie. Because of this :
- You should not store sensitive data on cookies.
- You should not store data that belongs to one user account.
- Cookie has no effect on server resources.
- The cookie expires at the specified date by mention in expire time.
Properties of HttpCookies Class
- Name: Contains the name of the Key.
- Domain: Using these properties we can set the domain of the cookie.
- Expires: This property sets the Expiration time of the cookies.
- HasKeys: If the cookies have a subkey then it returns True.
- Value: This contains the value of the cookies.
- Secured: If the cookies are to be passed in a secure connection then it only returns True.
- Path: Contains the Virtual Path to be submitted with the Cookies.
Add Information into cookies
public ActionResult cookies() { // Create the cookie object. HttpCookie cookie = new HttpCookie("CodeIsAll"); cookie["website"] = "codeisall.com"; // This cookie will remain for one month. cookie.Expires = DateTime.Now.AddMonths(1); // Add it to the current web response. Response.Cookies.Add(cookie); return View(); }
Retrieve Cookies information
HttpCookie cookieObj = Request.Cookies["CodeIsAll"]; string _websiteValue = cookieObj["website"];
Session
The session is temporary storage, It is used to store and retrieve the value for a particular time session.
The session is generally used to store the information temporarily, like when the user login we can save the basic information temporarily, so when we need them we don’t have to do database calls every time.
Another example of a session is, suppose you have one system where you log in and you want a user to log in after every 4 hours, then you can expire the session to make them log in again.
Each created session is stored in SessionStateItemCollection object.
public ActionResult session() { // Create the session. Session["website"] = "codeisall.com"; return View(); }
Get session value into view from the following syntax.
<div> @if(Session["website"]!=null) { <p>session value : @Session["website"]</p> } </div>
I hope this article will help you to understand how cookies and sessions can be used in MVC applications and also how beneficial they are to use. we will be happy to help you if you have any issues or queries. Comment your suggestions and issues and we will try to resolve them.