jQuery datatables in MVC
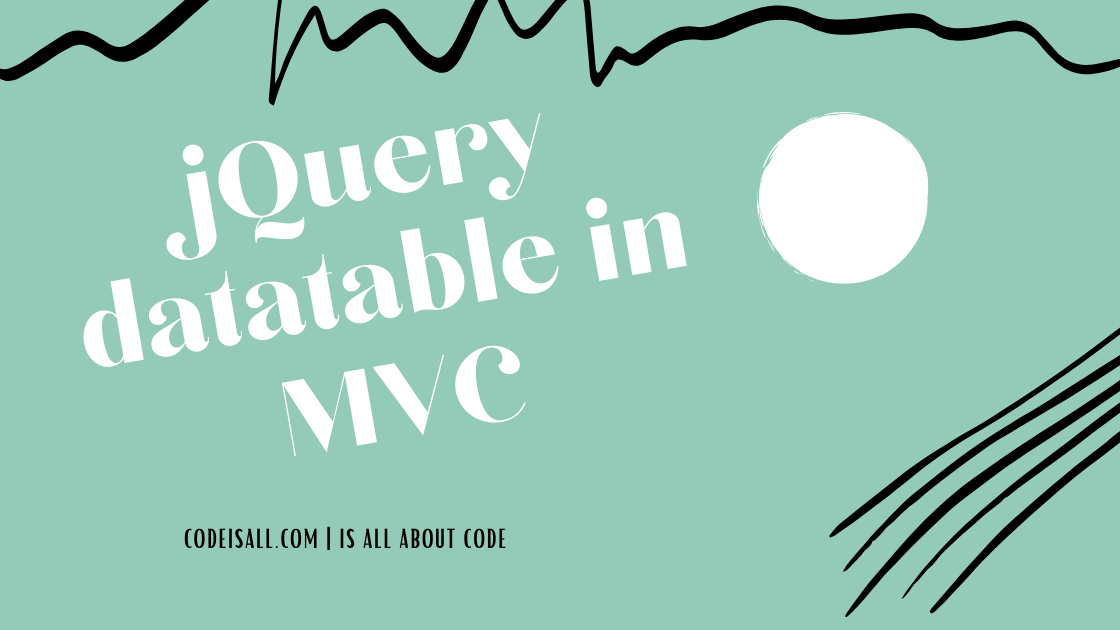
In the previous article, you learned about what is the Areas in MVC and how we can use them in MVC applications.Â
In this article, you will learn about what jQuery Datatables is, the advantages of jQuery Datatables, and how to use it in an MVC application.
What is jQuery Datatables
DataTables is a powerful jQuery plugin for creating tables in your web applications. It provides facilities for searching, sorting, and pagination without any configuration.
You can manage a large number of records in tabular form using the jQuery Datatable plugin with a custom configuration for page size, no of records, custom column sorting, etc.
Advantages of jQuery Datatables
- Free open-source software
- Zero configuration
- Pagination
- Instant search
- Multi-column ordering
- Use almost any data source
- Easily theme-able
- Wide variety of extensions
- Mobile friendly
- Alternative pagination styles
- Change language information
- And many more
Setting up jQuery Datatables
Download a stable version of jQuery Datatables from datatable’s official site. Place that download code into your project and use it. Otherwise, you can use CDN for the jQuery Datatables file. As the name suggests it is a jQuery plugin, you must include the jQuery library too from their official site.
jQuery Datatables in MVC Application
Step 1
Create a new project in asp.NET MVC, you can refer to create an asp.NET MVC application from here. Either you can also implement it in existing applications or projects as well.
Step 2
Download jQuery Datatables from here. Or you can use CDN as well and you can also use NuGet package manager for .NET applications. asp.NET project by default loaded jQuery and Bootstrap library, so no need to download jQuery library separately.
Step 3
Place the downloaded library in the Scripts folder or any other folder you like. As shown in the below image there are many js files located in the Scripts folder. And all are associated with jQuery and Bootstrap library files. I have put my downloaded jQuery Datatables into it.
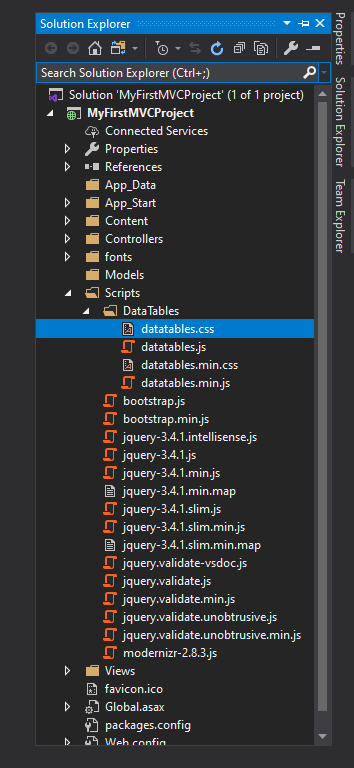
That contains CSS files for applying styles for datatable and js files for functions like sorting, searching, and pagination.
Step 4
Include jQuery Datatables library from _Layout.cshtml
the file or your view page. I’m including it on _Layout.cshtml
page so it’s globally accessible. Write the following line to include your datatable style to the web application. You should write it in before the </head>
tag.
<link href="~/Scripts/DataTables/datatables.css" rel="stylesheet">
Write another line to include your datatable js to the web application. You should write it in before the </body>
tag.
<script src="~/Scripts/DataTables/datatables.js"></script>
You can find there is some code block that looks like @Scripts.Render(“~/bundles/bootstrap”) that is the part of bundling, you can also add your stylesheet and js file into bundling. For that, you can refer to bundling and minification.
Step 5
I’m displaying data in a Home controller’s Index view. You can create a view for displaying data in data tables. For adding a new view go to the controller and add the method, right-click on the newly created method and add the view.
<div class="row"> <h1 class="text-center">jQuery Datatables using asp.NET</h1> <hr> <table id="example-table" class="display" style="width:100%"> <thead> <tr> <th>Name</th> <th>Position</th> <th>Start date</th> <th>Salary</th> </tr> </thead> <tbody> <tr> <td>Tiger Nixon</td> <td>System Architect</td> <td>2011/04/25</td> <td>320,800</td> </tr> <tr> <td>Garrett Winters</td> <td>Accountant</td> <td>2011/07/25</td> <td>170,750</td> </tr> <tr> <td>Ashton Cox</td> <td>Junior Technical Author</td> <td>2009/01/12</td> <td>86,000</td> </tr> <tr> <td>Cedric Kelly</td> <td>Senior Javascript Developer</td> <td>2012/03/29</td> <td>433,060</td> </tr> <tr> <td>Airi Satou</td> <td>Accountant</td> <td>2008/11/28</td> <td>162,700</td> </tr> <tr> <td>Brielle Williamson</td> <td>Integration Specialist</td> <td>2012/12/02</td> <td>372,000</td> </tr> <tr> <td>Herrod Chandler</td> <td>Sales Assistant</td> <td>2012/08/06</td> <td>137,500</td> </tr> <tr> <td>Rhona Davidson</td> <td>Integration Specialist</td> <td>2010/10/14</td> <td>327,900</td> </tr> <tr> <td>Colleen Hurst</td> <td>Javascript Developer</td> <td>2009/09/15</td> <td>205,500</td> </tr> <tr> <td>Sonya Frost</td> <td>Software Engineer</td> <td>2008/12/13</td> <td>103,600</td> </tr> <tr> <td>Jena Gaines</td> <td>Office Manager</td> <td>2008/12/19</td> <td>90,560</td> </tr> <tr> <td>Quinn Flynn</td> <td>Support Lead</td> <td>2013/03/03</td> <td>342,000</td> </tr> </tbody> </table> </div> @section scripts{ <script> $(document).ready(function () { $('#example-table').DataTable(); }); </script> }
Here in the above code apply datatable using table id selector. The above example is zero configuration you just need to call $().DataTable();
function.
searching, sorting, and pagination by default added to the table as shown in the example.
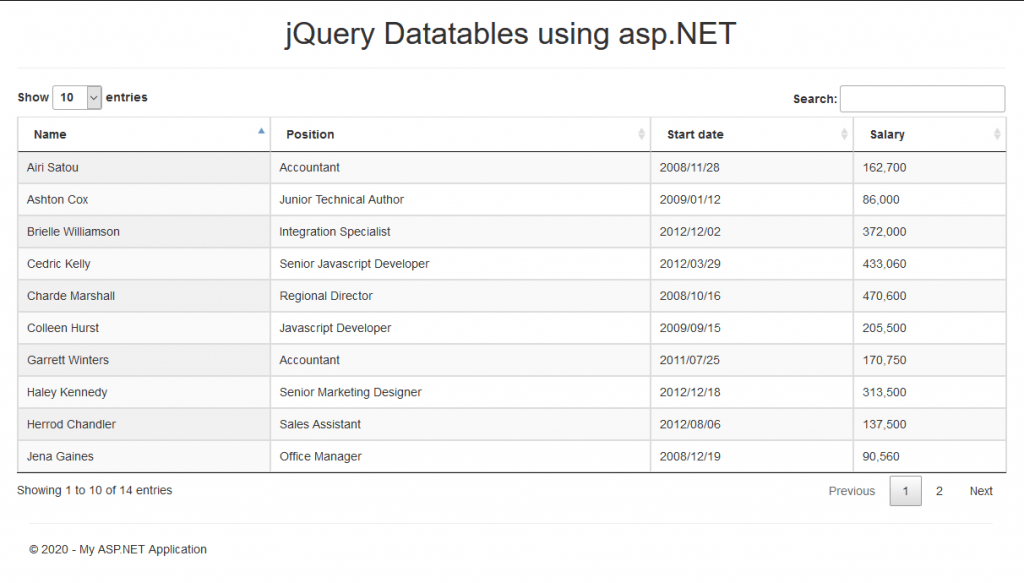
$('#table2').DataTable({"pageLength": 5});
From the following code block, I have set data size 5 per page. If you are not writing pageLength then it takes 10 by default.
Binding Data with Array
You can bind datatable data with an array. You write just table head or just table and set the data property to the array name. The following example shows binding data with arrays.
<div class="row"> <h1 class="text-center">jQuery Datatables using asp.NET</h1> <h3 class="text-center">Binding Data with Array</h3> <hr> <table id="example-table" class="display" style="width:100%"> <thead> <tr> <th>Name</th> <th>Position</th> <th>Start date</th> <th>Salary</th> </tr> </thead> </table> </div> @section scripts{ <script> var tableData = [ [ "Tiger Nixon", "System Architect", "2011/04/25", "320,800" ], [ "Garrett Winters", "Accountant", "2011/07/25", "170,750" ], [ "Ashton Cox", "Junior Technical Author", "2009/01/12", "86,000" ], [ "Cedric Kelly", "Senior Javascript Developer", "2012/03/29", "433,060" ], [ "Airi Satou", "Accountant", "2008/11/28", "162,700" ], [ "Brielle Williamson", "Integration Specialist", "2012/12/02", "372,000" ], [ "Herrod Chandler", "Sales Assistant", "2012/08/06", "137,500" ], [ "Rhona Davidson", "Integration Specialist", "2010/10/14", "327,900" ], [ "Colleen Hurst", "Javascript Developer", "2009/09/15", "205,500" ], [ "Sonya Frost", "Software Engineer", "2008/12/13", "103,600" ], [ "Jena Gaines", "Office Manager", "2008/12/19", "90,560" ], [ "Quinn Flynn", "Support Lead", "2013/03/03", "342,000" ], [ "Charde Marshall", "Regional Director", "2008/10/16", "470,600" ], [ "Haley Kennedy", "Senior Marketing Designer", "2012/12/18", "313,500" ] ]; $(document).ready(function () { $('#example-table').DataTable({ data: tableData, "pageLength": 5 }); }); </script> }
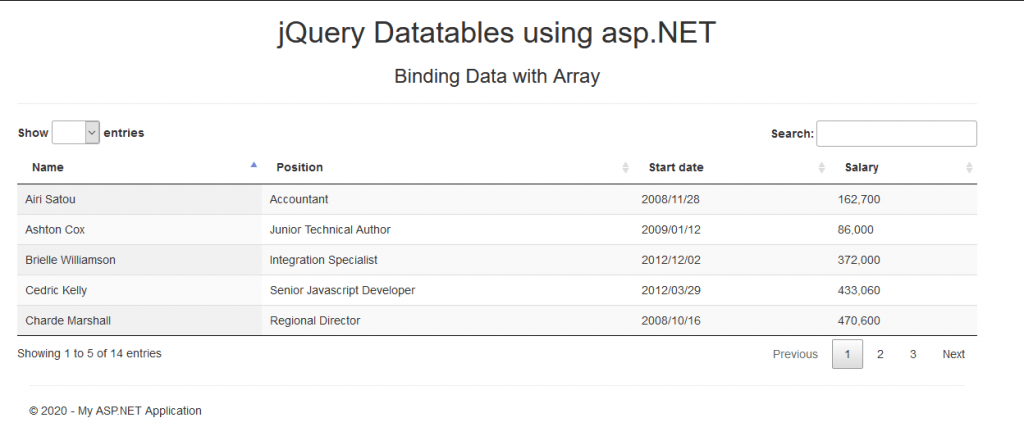
Binding Data with JSON
In the above example, we are binding data with an array, we can also set data using JSON as well, you have to set the fields of the JSON to the columns property of DataTables.
<div class="row"> <h1 class="text-center">jQuery Datatables using asp.NET</h1> <h3 class="text-center">Binding Data with JSON</h3> <hr> <table id="example-table" class="display" style="width:100%"> <thead> <tr> <th>Name</th> <th>Position</th> <th>Start date</th> <th>Salary</th> </tr> </thead> </table> </div> @section scripts{ <script> var tableDataJson = [ { "Name": "Tiger Nixon", "Position": "System Architect", "StartDate": "2011/04/25", "Salary": "320,800" }, { "Name": "Garrett Winters", "Position": "Accountant", "StartDate": "2011/07/25", "Salary": "170,750" }, { "Name": "Ashton Cox", "Position": "Junior Technical Author", "StartDate": "2009/01/12", "Salary": "86,000" }, { "Name": "Cedric Kelly", "Position": "Senior Javascript Developer", "StartDate": "2012/03/29", "Salary": "433,060" }, { "Name": "Airi Satou", "Position": "Accountant", "StartDate": "2008/11/28", "Salary": "162,700" }, { "Name": "Brielle Williamson", "Position": "Integration Specialist", "StartDate": "2012/12/02", "Salary": "372,000" }, { "Name": "Herrod Chandler", "Position": "Sales Assistant", "StartDate": "2012/08/06", "Salary": "137,500" }, { "Name": "Rhona Davidson", "Position": "Integration Specialist", "StartDate": "2010/10/14", "Salary": "327,900" }, { "Name": "Colleen Hurst", "Position": "Javascript Developer", "StartDate": "2009/09/15", "Salary": "205,500" }, { "Name": "Sonya Frost", "Position": "Software Engineer", "StartDate": "2008/12/13", "Salary": "103,600" }, { "Name": "Jena Gaines", "Position": "Office Manager", "StartDate": "2008/12/19", "Salary": "90,560" }, { "Name": "Quinn Flynn", "Position": "Support Lead", "StartDate": "2013/03/03", "Salary": "342,000" }, { "Name": "Charde Marshall", "Position": "Regional Director", "StartDate": "2008/10/16", "Salary": "470,600" }, { "Name": "Haley Kennedy", "Position": "Senior Marketing Designer", "StartDate": "2012/12/18", "Salary": "313,500" } ]; $(document).ready(function () { $('#example-table').DataTable({ data: tableDataJson, columns: [ { data: 'Name' }, { data: 'Position' }, { data: 'StartDate' }, { data: 'Salary' } ], "pageLength": 5 }); }); </script> }
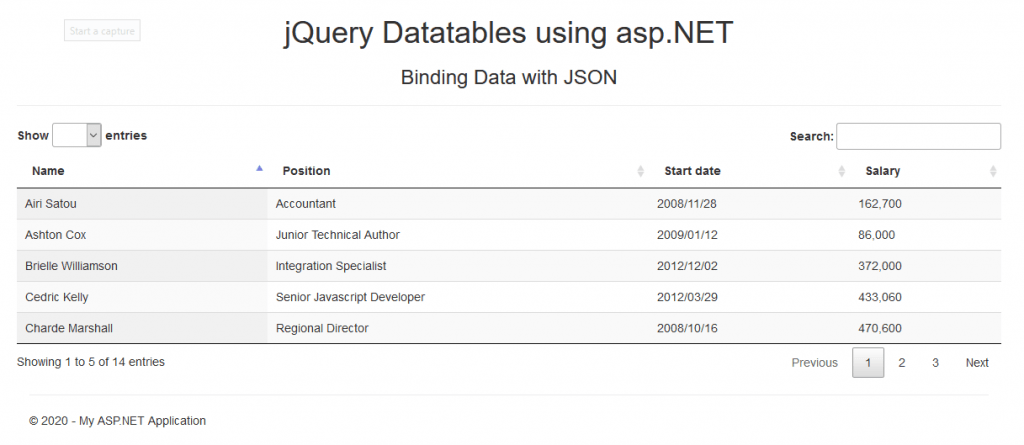
Binding with AJAX Request
In jQuery datatable you can set data from JSON data sources like files or APIs and set it into datatable using an AJAX request. Here I use an AJAX request to get data from the JSON file that is located in my project’s Content folder. Make an AJAX call to get data from the JSON file and show that data into datatable.
EmpDataSource.json
{ "EmpsData": [{ "Name": "Tiger Nixon", "Position": "System Architect", "StartDate": "2011/04/25", "Salary": "320,800" }, { "Name": "Garrett Winters", "Position": "Accountant", "StartDate": "2011/07/25", "Salary": "170,750" }, { "Name": "Ashton Cox", "Position": "Junior Technical Author", "StartDate": "2009/01/12", "Salary": "86,000" }, { "Name": "Cedric Kelly", "Position": "Senior Javascript Developer", "StartDate": "2012/03/29", "Salary": "433,060" }, { "Name": "Airi Satou", "Position": "Accountant", "StartDate": "2008/11/28", "Salary": "162,700" }, { "Name": "Brielle Williamson", "Position": "Integration Specialist", "StartDate": "2012/12/02", "Salary": "372,000" }, { "Name": "Herrod Chandler", "Position": "Sales Assistant", "StartDate": "2012/08/06", "Salary": "137,500" }, { "Name": "Rhona Davidson", "Position": "Integration Specialist", "StartDate": "2010/10/14", "Salary": "327,900" }, { "Name": "Colleen Hurst", "Position": "Javascript Developer", "StartDate": "2009/09/15", "Salary": "205,500" }, { "Name": "Sonya Frost", "Position": "Software Engineer", "StartDate": "2008/12/13", "Salary": "103,600" }, { "Name": "Jena Gaines", "Position": "Office Manager", "StartDate": "2008/12/19", "Salary": "90,560" }, { "Name": "Quinn Flynn", "Position": "Support Lead", "StartDate": "2013/03/03", "Salary": "342,000" }, { "Name": "Charde Marshall", "Position": "Regional Director", "StartDate": "2008/10/16", "Salary": "470,600" }, { "Name": "Haley Kennedy", "Position": "Senior Marketing Designer", "StartDate": "2012/12/18", "Salary": "313,500" } ] }
View File
<div class="row"> <h1 class="text-center">jQuery Datatables using asp.NET</h1> <h3 class="text-center">Binding Data with AJAX</h3> <hr> <table id="example-table" class="display" style="width:100%"> <thead> <tr> <th>Name</th> <th>Position</th> <th>Start date</th> <th>Salary</th> </tr> </thead> </table> </div> @section scripts{ <script> $(document).ready(function () { $('#example-table').DataTable({ ajax: { url: "https://localhost:44367/Content/EmpDataSource.json", dataSrc: "EmpsData" }, columns: [ { data: 'Name' }, { data: 'Position' }, { data: 'StartDate' }, { data: 'Salary' } ], "pageLength": 5 }); }); </script> }
To make AJAX requests you need to use an AJAX property of datatable and provide URL and dataSrc to that property.
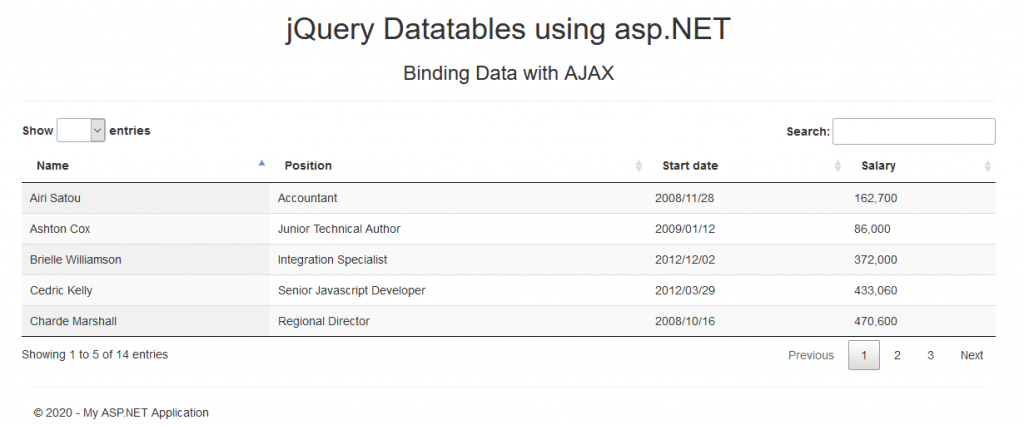
You can find more examples and advanced options on datatable official site.
I hope this article will help you to understand how jQuery Datatables can be used in MVC applications and also what is the advantages of using jQuery Datatables. We will be happy to help you if you have any issues or queries. Comment your suggestions and issues and we will try to resolve them.