How to use Dapper ORM in C#
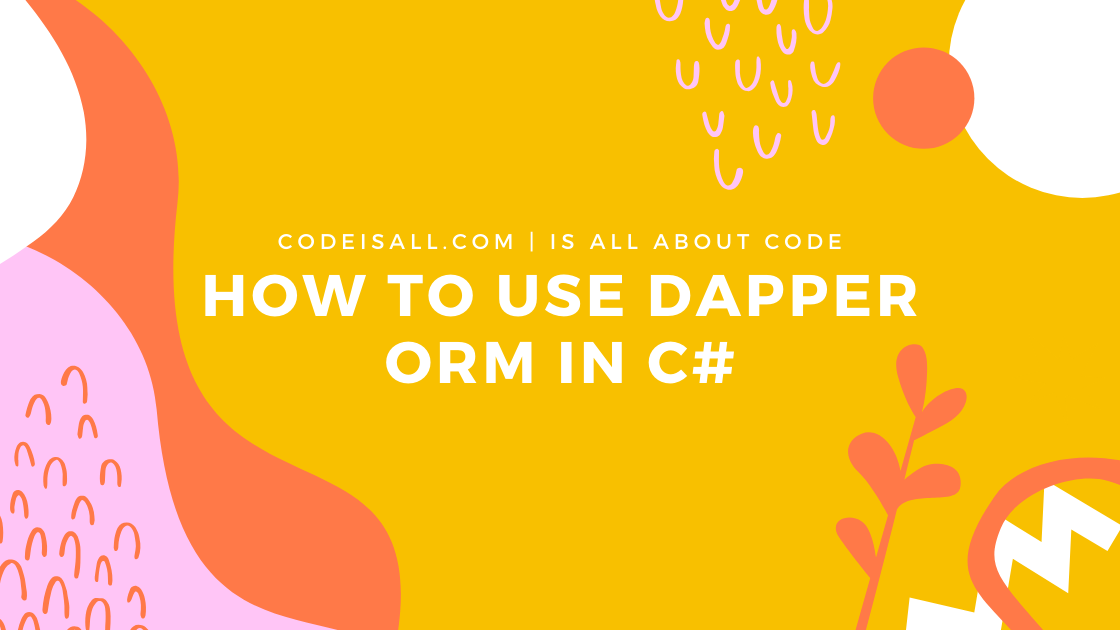
Introduction
In the previous article, you have learned about what is ViewBag , ViewData, and TempData, their scope, and how to use it in the MVC application.
In this article, you will learn how to map the database data to the model and display it in a view with Dapper Micro ORM.
This article will be helpful to beginners and students who are learning MVC.
What is Dapper
Basically, Dapper is a micro ORM , It is a simple object mapper framework that helps to map the database query output to the domain class. It’s a open source and high perfomance system.
It extends the IDbConnection interface. The IDbConnection interface represents an open connection to data source implemented by the .NET framework.
If your MVC app contains store procedure or native query instead of using ORM tool like entity-framework, then it’s preferable to use the Micro ORM like dapper.
Pre-Requisites
- You need to create ASP.NET MVC Project(You can refer our Create Asp .Net MVC application article for that), Or you can add this into the existing project also A SQL server Installed where you can create a database.
- A Database created on the SQL server.
Implementation
Step 1:
In the project add the nu-get package named “Dapper”. To install Dapper open a package manager and run the below code, it will take a few seconds to install.
Install-Package Dapper
Step 2:
Create a model class that has the exact same property as the database. In our case replace the below-given code.
User.cs
public class User { public int Id { get; set; } public string FirstName { get; set; } public string LastName { get; set; } public string Email { get; set; } public string PhoneNumber { get; set; } public string Password { get; set; } }
Step 3:
Add the below code to the method that returns the list of data from database to the view,
Here we have index method from Home controller which returns the data of user table in the view page of the Index action that Index.cshtml
Add the below given code of the Index.cshtml and Index action of Home.cs
In Index action we are using IDbConnection to connect to the database, and we are fetching data from the Users table.
Home.cs
public class HomeController : Controller { public ActionResult Index() { List userList = new List(); using (IDbConnection db = new SqlConnection("server=Niksart; Initial Catalog=DemoDB;Integrated Security=true")) { userList = db.Query("Select * From Users").ToList(); } return View(userList); } }
Index.cshtml
And when you the code it will display the records of User table as shown below in the browser.
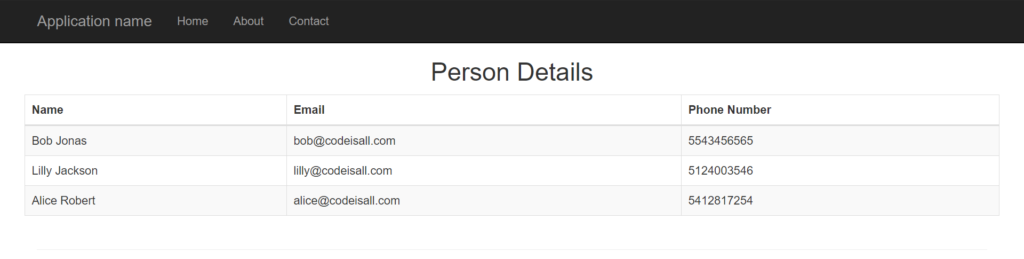
I hope this article will help you to fetch data from the database with the Dapper mapping in the MVC application. we will be happy to help you if you have any issues or queries. Comment your suggestions and issues and we will try to resolve it.