Android Login Screen Design
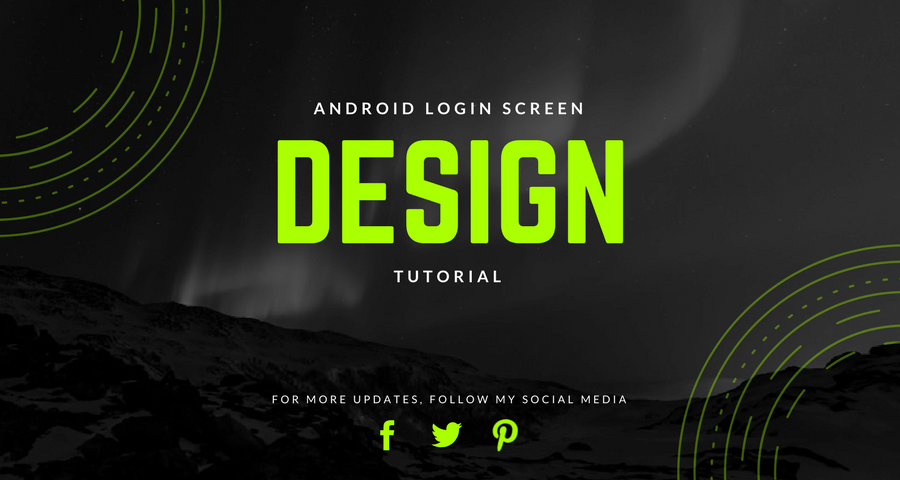
Hello, welcome to android login screen design tutorial. In this tutorial, we are going to design a login screen with the help of custom drawable resources and android studio, so without wasting time let’s start with the tutorial.
First of all, create a new project in the android studio, and give appropriate details like project name, package name etc. Then select Empty Activity and give your activity name and click finish.
Now go to res/values/styles.xml and make changes like following.
styles.xml
<resources> <!-- Base application theme. --> <style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar"> <!-- Customize your theme here. --> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> </style> </resources>
Now create a new drawable resource. (right click on drawable folder select New > Drawable resource file).
rounded_edit_text.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item> <shape android:shape="rectangle"> <solid android:color="#00000000"/> <corners android:radius="30dp" /> <stroke android:width="1dp" android:color="#FFFFFF" /> </shape> </item> </selector>
Create another drawable file for the button.
rounded_button.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item> <shape android:shape="rectangle"> <solid android:color="#689f38"/> <corners android:radius="30dp" /> </shape> </item> </selector>
I’m using a background image if you want same background image you can download it from the following link and paste it in drawable folder.
Click Here To Download Background Image
Now make our activity layout file like following
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:gravity="center" android:background="@drawable/background" android:orientation="vertical" android:layout_height="match_parent" tools:context="com.codeisall.androidloginscreen.MainActivity"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Welcome" android:textColor="#FFFFFF" android:gravity="center" android:textSize="45sp"/> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="To access all features of the app provided by Code Is All, You need to login first." android:textColor="#FFFFFF" android:gravity="center" android:textSize="12sp" android:layout_marginTop="10dp" android:layout_marginBottom="10dp" android:layout_marginLeft="30dp" android:layout_marginRight="35dp"/> <EditText android:layout_width="300dp" android:layout_height="40dp" android:background="@drawable/rounded_edit_text" android:hint="Email Address" android:layout_margin="10dp" android:inputType="text" android:textColor="#000000" android:textColorHint="#FFFFFF" android:gravity="center"/> <EditText android:layout_width="300dp" android:layout_height="40dp" android:background="@drawable/rounded_edit_text" android:text="123456789" android:textColor="#FFFFFF" android:layout_margin="10dp" android:inputType="textPassword" android:textColorHint="#FFFFFF" android:gravity="center"/> <Button android:layout_width="300dp" android:layout_height="40dp" android:layout_margin="10dp" android:text="Login" android:textColor="#FFFFFF" android:background="@drawable/rounded_button"/> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="horizontal"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textColor="#FFFFFF" android:textSize="14sp" android:text="Forgot Password"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textColor="#FFFFFF" android:textSize="14sp" android:text=" | "/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textColor="#FFFFFF" android:textSize="14sp" android:text="Register"/> </LinearLayout> </LinearLayout>
Now open your MainActivity.java file and add two lines of code as shown below. So when we launch our application, our activity opened as a full-screen activity.
MainActivity.java
package com.codeisall.androidloginscreen; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.Window; import android.view.WindowManager; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); requestWindowFeature(Window.FEATURE_NO_TITLE); getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN); setContentView(R.layout.activity_main); } }
Now, we can launch our application, the following is the output.
please expain your code
1. button are transparent.etc
and more explinaton about the steps
Visitor Rating: 5 Stars
Visitor Rating: 5 Stars
Visitor Rating: 5 Stars
Visitor Rating: 5 Stars